How to read, write, append and create TEXT files in Python, including how to read text files line by line
Links to topics on this page
- Working with files in Python
- Two ways to compile the open() function statement in Python
- Open files in the same or different directory
- Read a text file in Python using read() or readlines()
- Read text from a file in Python and save (write) to another file
- open() function arguments
Working with files in Python
Use the base function open()
to handle files in Python.
Two arguments file
and mode
will cover most simple cases of reading and writing to text files.
open(file = , mode = )
There are five modes relating to file actions:
- “r” – read the file
- “w” – write to the file (overwrite existing content)
- “a” – append to the file
- “x” – create a new file (will return an error if the file already exists)
- “+” – open for updating – reading and writing.
The first 3 modes above (r, w and a) create a new file if the file doesn’t already exist. Note also that the mode argument is entered as a “string“ value.
In addition, there are two other modes ‘b’ for binary and ‘t’ for text (the default). If you set mode to ‘b’ the file will be read in binary mode.
Open files in the same or different directory – file path for the text file
The file argument takes the name of a file as a string value – just the file name if the file is in the same directory as the script; include the relative file reference in the file path if the file is located in a different directory.
# opens and reads the file named 'my_text_file' in the same directory as this script
open("my_text_file", "r")
# opens and reads the file named 'my_text_file' in the sub-folder 'files'
open("./files/my_text_file", "r")
Note that Python does all the text processing itself, and doesn’t rely on the underlying operating system’s handling of text files.
Two ways to compile the open() function statement in Python
Choose between two different ways to structure a statement using the open() function in Python, depending on other code steps that you want to perform.
1. Create an object in a single statement
Use the open()
command to create an object which is then operated on in a separate statement. In the example below, the object f
is created which opens the text file in read mode. In a separate statement the text is then read from the file and loaded into the object ‘text_from_file’, using the .read()
function. This method should include the .close() function afterwards otherwise the file will remain open, potentially using up valuable resources.
f = open("wordle_words.txt", "r")
text_from_file = f.read()
# with this method you need to close the file object
f.close()
2. Use with
and open()
for adding further code steps
In this structure, the command with
is used to create the file object and then additional code steps can follow. In the example below, the first line sets up the object f_new2
with open file and mode, followed by the .write()
function. Another benefit of this method is that the with
block automatically closes the target file when focus moves from the code block, so the close() function doesn’t need to be called explicitly.
# this method will automatically close the file afterwards
with open ("./files/new_text_file", "w") as f_new2:
f_new2.write(text_from_file)
Read a text file in Python using read() or readlines()
The output is different depending on whether .read() or .readlines() is used.
Using read()
to read in text – output is in string format
# open the file named 'wordle_words.txt' in read mode
f = open("wordle_words.txt", "r")
# read and load the text from the file and save in object 'text_from_file'
text_from_file = f.read()
# this creates an object of type str()
type(text_from_file)
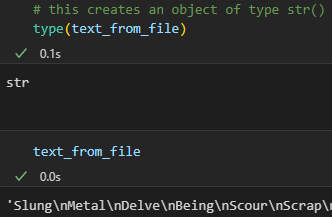
Using readlines()
to read in text – output is in list format
# open the file named 'wordle_words.txt' in read mode
f = open("wordle_words.txt", "r")
# read and load the text from the file and save in object 'text_from_file'
text_from_file = f.readlines()
# this creates an object of type list()
type(text_from_file)
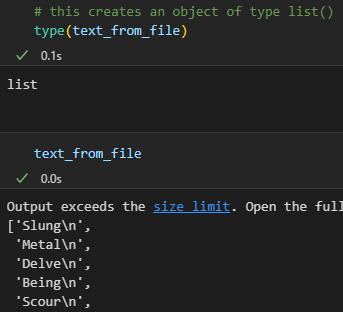
Read text from a file in Python and save (write or append) to another file
We may want to open a text file as a template with common code, and then save it under another file name, e.g. to append other text to it. In the example below we could also have used the ‘with’ structure in the first step of reading in the text file. Additionally, you could also have the second file already created with content, and append the read-in text to the end.
# open the file named 'wordle_words.txt' in read mode
f = open("wordle_words.txt", "r")
# read and load the text from the file and save in object 'text_from_file'
text_from_file = f.read()
# this creates an object of type str()
type(text_from_file)
# write the text to a file named 'new_text_file' using the write mode
# this will create a new file if it doesn't already exist, or
# overwrite the content of a file that does exist
with open ("new_text_file.txt", "w") as f_new:
f_new.write(text_from_file)
# close the initial file because we didn't use the 'with' method in the first step
f.close()
open() function arguments
The full list of open()
function arguments is:
open(file, mode='r', buffering=- 1, encoding=None, errors=None, newline=None, closefd=True, opener=None)
Links to related topics
- Python built-in functions –
open()
https://docs.python.org/3/library/functions.html#open - Python relative import and dynamic file paths